There are many free and commercial chart libraries that can be used in React. Examples are:
React Chart: https://react-charts.tanstack.com/
Recharts: https://recharts.org/en-US
Nivo : https://nivo.rocks/
Highcharts : https://www.highcharts.com/integrations/react/
Charts can be used to visually represent and analyze datasets, providing intuitive and interactive data visualization for better understanding. Whether displaying trends over time, comparing multiple datasets, or showcasing statistical information, charts offer a dynamic way to convey information.
In this tutorial, we use Recharts.
Step 1. Create a new React App (using VS code)
If you are using a React online editor, you can proceed to step 2.
Create a new project in VS code.
Step 2. Add Recharts library to the project
Recharts is a third-party charting library for React and is not a part of the React core. To integrate Recharts into your project, open a terminal window within your project directory and install it by typing the following command. In online editors, you can achieve the same by entering the command in the specific section where you manage libraries.
npm install recharts
To ensure that you have installed it correctly, you can check your package.json file in the root folder of your project, and you should find ‘recharts’ listed in the dependencies section.
Step 3. Remove extra code from App.jsx
In this step, remove the default extra code from ‘App.jsx’ and import ‘useState’ and ‘useEffect.’ Utilize ‘useState’ to create component state and store the data, while ‘useEffect’ will handle data fetching. Locate the ‘App.jsx’ file in the ‘src’ folder and modify it as follows:
import React, { useEffect, useState } from "react";
import './App.css'
function App() {
return (
<>
</>
)
}
export default App
Step 4. fetch the data
In this step, we need to fetch the data that will be displayed on the Line Chart. For more information see “how to fetch data in React using API or JSON”.
Add line 7-17 to the App function. The code fetch the data from JSON file and store data in a component state co2data. You can download the JSON file here.
import { Co2LineChart } from "./Co2LineChart";
import { useState, useEffect } from 'react'
import './App.css'
function App() {
const [co2data, setCo2data] = useState([]);
useEffect(() => {
setCo2data([]);
const url = "CO2EmissionsData.json"; //do not forget to copy CO2EmissionsData.json to the public folder
fetch(url)
.then(data => data.json())
.then(data => {
setCo2data(data);
});
}, [])
return (
<>
</>
)
}
export default App
Step 5. Adding the Co2LineChart component
Add a new file in the source folder “Co2LineChart.jsx” and create a new component with the same name.
export function Co2LineChart({ myData }) {
return (
<>
</>
)
}
{myData}
represents the data that we will later send to this component to be displayed on the line chart. Therefore, it serves as the component input here.
Another option to create a new component is to use an arrow function when creating the component; both approaches achieve the same result.
export const Co2LineChart = ({ myData }) => {
return (
<>
</>
)
}
Do not forget to import this component in the parent component (App) using the following line. You can add it to the start of the App.jsx:
import { Co2LineChart } from "./Co2LineChart";
Also, add it to the reture section of the App component by using the following code:
<>
{co2data.length > 0 &&
<Co2LineChart myData={co2data}></Co2LineChart>
}
</>
Since the data fetching is an asynchronous operation, line 2 checks to render the component when the data is ready. If data is null, component is not rendered.
Step 6. Importing and adding the line chart from Recharts
To import the line chart from Recharts, include the following important command at the beginning of your Co2LineChart.jsx
file. To incorporate a line chart into your app, use the following code with <LineChart>
and the same parameters. Detailed information on importing and utilizing the line chart can be found on the Recharts website. Now, Co2LineChart.jsx
should look like this.
import {
LineChart,
Line,
XAxis,
YAxis,
CartesianGrid,
Tooltip,
Legend
} from "recharts";
export const Co2LineChart = ({ myData }) => {
return (
<div >
<LineChart
width={1000}
height={500}
data={myData}
margin={{
top: 50,
right: 30,
left: 50,
bottom: 5
}}
>
<CartesianGrid strokeDasharray="3 3" />
<XAxis dataKey="Year" />
<YAxis />
<Tooltip />
<Legend />
<Line
type="monotone"
dataKey="Total"
stroke="#8884d8"
activeDot={{ r: 8 }}
/>
</LineChart>
</div >
)
}
To specify which dataset the chart should use, you can use data={myData}
inside the <LineChart>
.
The x-axis of the chart always shows the ‘Year’ data from the dataset. This is done by setting the ‘dataKey’ property in <XAxis>
.
<Line>
is used to add a line to the chart. The ‘dataKey’ property inside it specifies which part of the data you want to show on the graph. In this example, we added one line which always shows the total value from the dataset. We will change this later to make it more dynamic.
Step 7. Make the chart dynamic
We want to add another line to the chart to display an additional value from the dataset alongside the Total line we’ve already added. To achieve this, add a dropdown element before the chart with the following values:
<label>
Pick type of data:
<select
value={selectedData}
onChange={onDataChange}
>
<option value="Gas Fuel">Gas Fuel</option>
<option value="Liquid Fuel">Liquid Fuel</option>
<option value="Solid Fuel">Solid Fuel</option>
<option value="Cement">Cement</option>
<option value="Gas Flaring">Gas Flaring</option>
</select>
</label>
We also need an event handler to capture the user’s selection and display the relevant data on the graph. For example, if the user chooses ‘Solid Fuel,’ we should show two lines on the graph: one representing the total, which is independent of the user’s selection, and the other representing the user’s selection, which is ‘Solid Fuel.’ This allows for a comparison between the total CO2 emission value and the CO2 emission specifically from solid fuels. The user selection trigger this event in the dropdown:
onChange={onDataChange}
We also need to add a component state to store the user’s selection by adding the following code to your Co2LineChart
component. The code also includes the event handler that captures the user’s selection and stores it in the component state.
const [selectedData, setSelectedData] = useState("Cement");
const onDataChange = (e) => {
setSelectedData(e.target.value);
}
Don’t forget to add useState
to the imports since the code above uses useState
:
import { useState } from "react";
Add a new line to your chart component you should include the following code at the end of <LineChart>
. Please note that the ‘dataKey’ in the new line is set to {selectedData}
in order to make the chart dynamic and utilize the selected value from the dropdown list.
<Line
type="monotone"
dataKey={selectedData}
stroke="#82ca9d"
/>
Step 8. Test the app
Now the Co2LineChart.jsx looks like this.
import { useState } from "react";
import {
LineChart,
Line,
XAxis,
YAxis,
CartesianGrid,
Tooltip,
Legend
} from "recharts";
export const Co2LineChart = ({ myData }) => {
const [selectedData, setSelectedData] = useState("Cement");
const onDataChange = (e) => {
setSelectedData(e.target.value);
}
return (
<div >
<label>
Pick type of data:
<select
value={selectedData}
onChange={onDataChange}
>
<option value="Gas Fuel">Gas Fuel</option>
<option value="Liquid Fuel">Liquid Fuel</option>
<option value="Solid Fuel">Solid Fuel</option>
<option value="Cement">Cement</option>
<option value="Gas Flaring">Gas Flaring</option>
</select>
</label>
<LineChart
width={1000}
height={500}
data={myData}
margin={{
top: 50,
right: 30,
left: 50,
bottom: 5
}}
>
<CartesianGrid strokeDasharray="3 3" />
<XAxis dataKey="Year" />
<YAxis />
<Tooltip />
<Legend />
<Line
type="monotone"
dataKey="Total"
stroke="#8884d8"
activeDot={{ r: 8 }}
/>
<Line
type="monotone"
dataKey={selectedData}
stroke="#82ca9d"
/>
</LineChart>
</div >
)
}
The output of the app should look like the following:
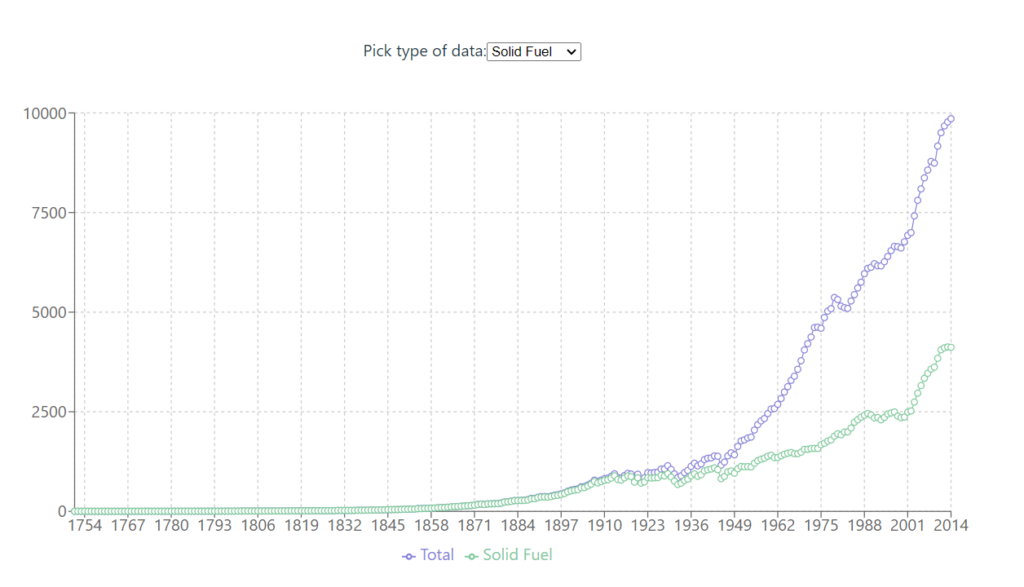
You can download the source code of the app here. Don’t forget to run ‘npm install’ before using ‘npm run dev’ to ensure all dependencies are installed.”